B.Error
题目描述:
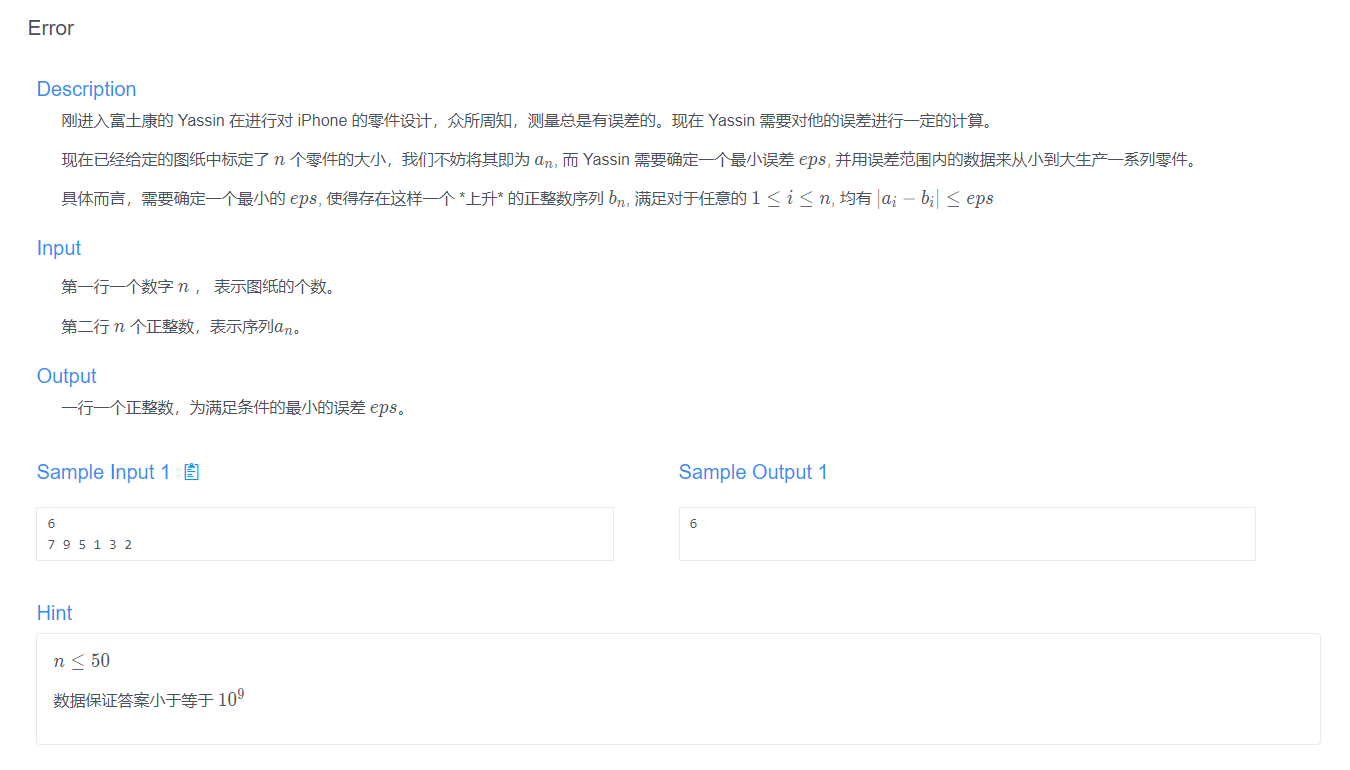
解题思路:
思维。问题可以转换为遍历计算 $max\lceil [(j - i) - (a[j] - a[i])] / 2.0 \rceil$
代码展示:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
| #include <bits/stdc++.h> using namespace std; typedef long long ll; const int mod=1e9+7; const int inf=0x7f7f7f7f; const int maxn=1e6+50;
int n, a[55], eps = 0;
int main(){ cin>>n; for(int i = 0; i < n; i++){ cin>>a[i]; }
for(int i = 0; i < n; i++){ for(int j = i + 1; j < n; j++){ int x = (j - i) - (a[j] - a[i]); eps = max(eps, (int)ceil(x / 2.0)); } } cout<<eps<<endl; return 0; }
|
E.吃利息
题目描述:
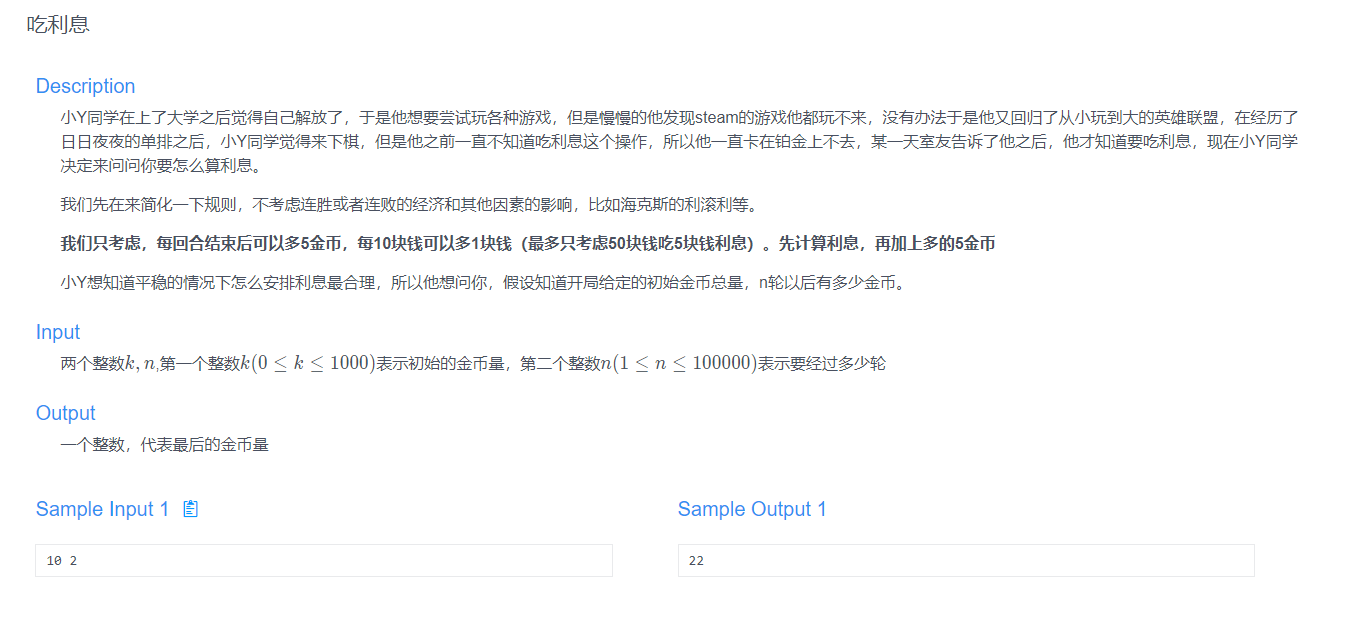
解题思路:
模拟每个回合。
代码展示:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| #include <bits/stdc++.h> using namespace std; typedef long long ll; const int mod=1e9+7; const int inf=0x7f7f7f7f; const int maxn=1e6+50;
int k,n;
int main(){ cin>>k>>n; for(int i = 0; i < n; i++){ k = k + min(k / 10, 5) + 5; }
cout<<k<<endl; return 0; }
|
G.MP4
题目描述:
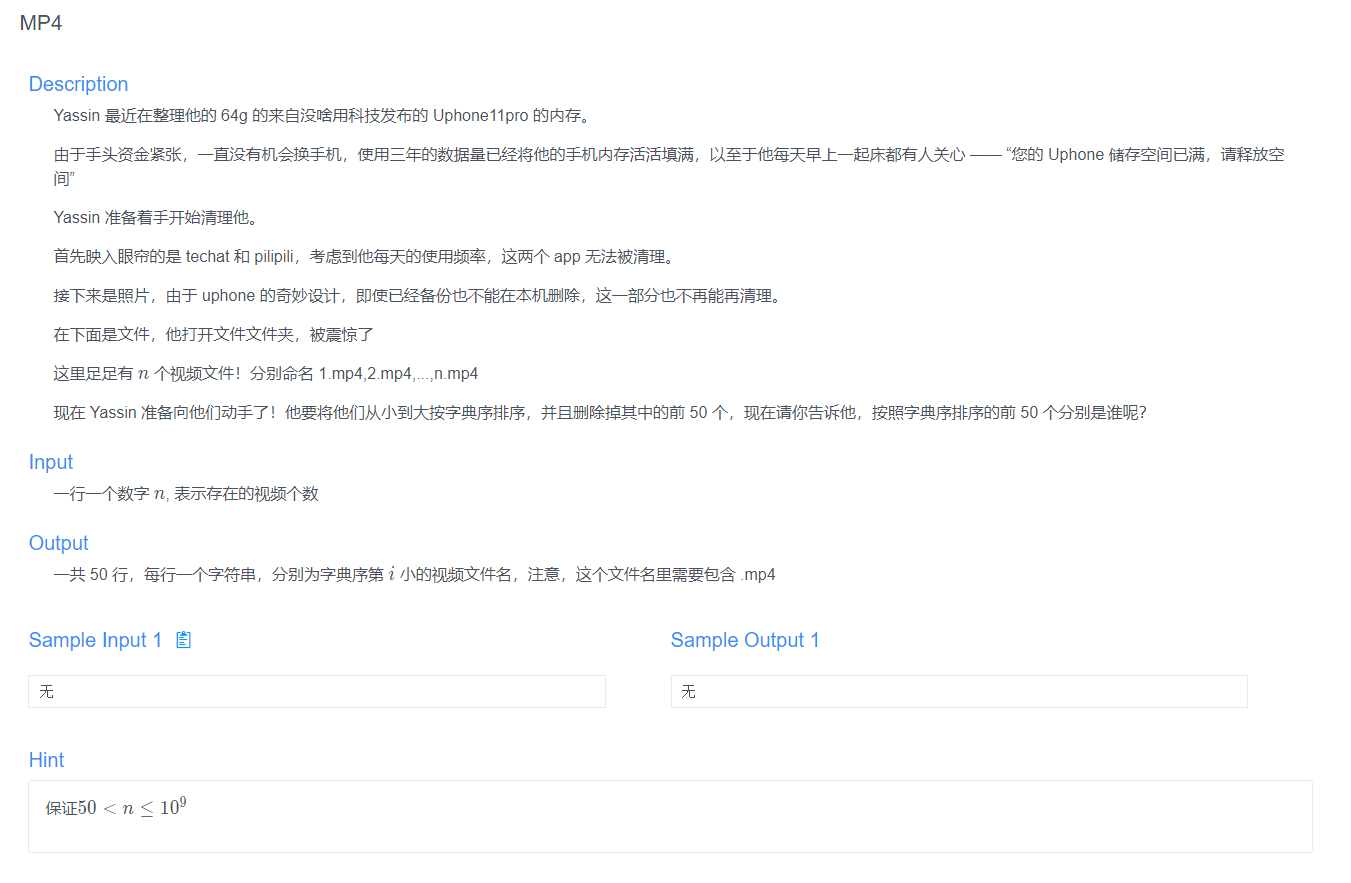
解题思路:
DFS。还可以直接转成字符串数组,对字符串数组按字典序排序。
代码展示:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40
| #include <bits/stdc++.h> using namespace std; typedef long long ll; const int mod=1e9+7; const int inf=0x7f7f7f7f; const int maxn=1e6+50;
int n, k = 50, digits = 0;; char s[55]; vector<string> ans;
void dfs(int n, int id){ if(k <= 0) return; if(id >= digits) return; for(int j = 0; j < 10; j++){ s[id] = j + '0'; s[id + 1] = '\0'; int x = atoi(s); if(x <= n && x > 0 && k){ --k; ans.push_back(s); dfs(n, id + 1); } } }
int main(){ cin>>n; int x = n; while(x){ x /= 10; ++digits; } dfs(n, 0); for(string s : ans){ cout<<s<<".mp4"<<endl; } return 0; }
|
I.展览
题目描述:
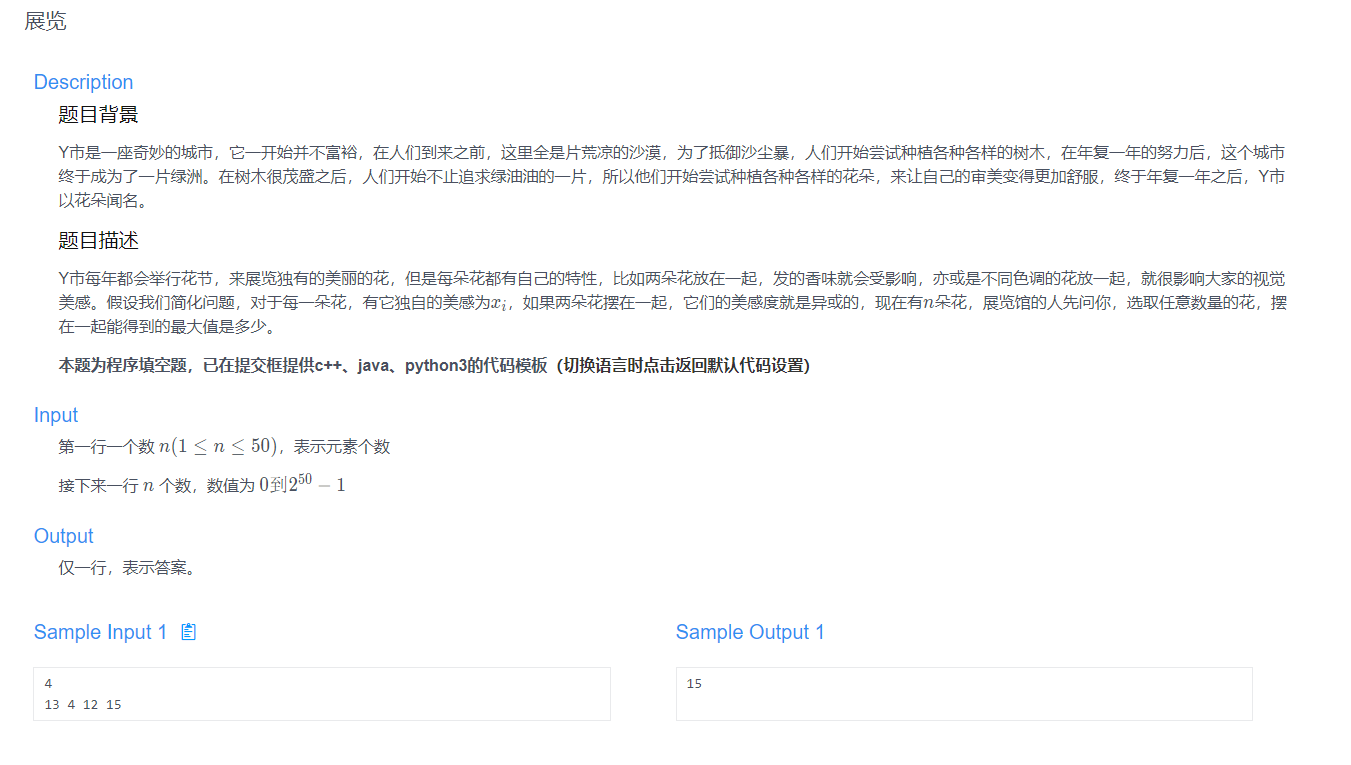
解题思路:
读代码注释填空,主要就是贪心+异或。
代码展示:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29
| #include<bits/stdc++.h> #define ll long long using namespace std; const ll N=1e6+5; ll n,b[N],a[N],ans;
void update(ll x){ for(ll i=60;i>=0;i--){ if((x&(1ll<<i))){ if(b[i])x^=b[i]; else {b[i] = x ;break;} } } }
int main(){ cin>>n; for(ll i=1;i<=n;i++){cin>>a[i];update(a[i]);} for(ll i=60;i>=0;i--)if((ans^(1ll<<i))>ans)ans^=b[i]; cout<<ans<<endl; return 0; }
|
K.礼物
题目描述:
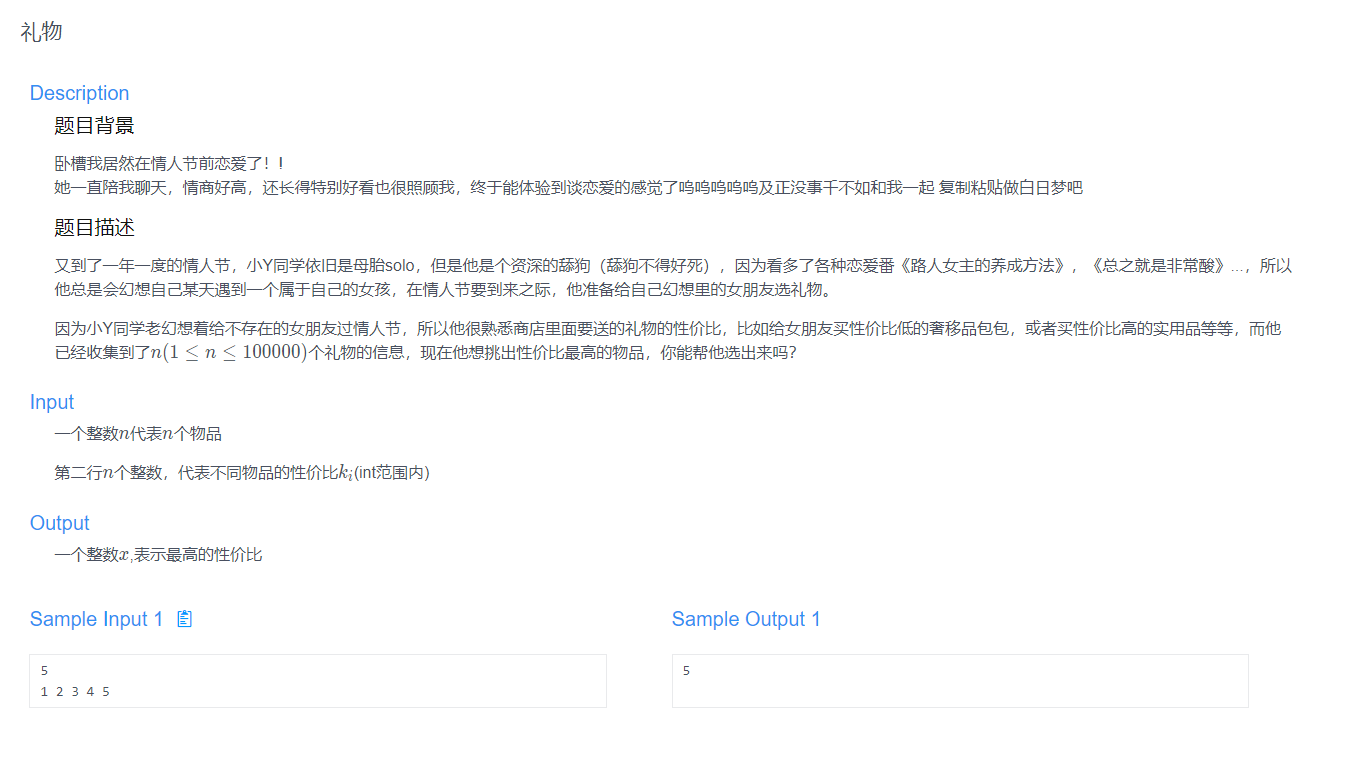
解题思路:
找最大。
代码展示:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| #include <bits/stdc++.h> using namespace std; typedef long long ll; const int mod=1e9+7; const int inf=0x7f7f7f7f; const int maxn=1e6+50;
int n, maxv = INT_MIN, x;
int main(){ cin>>n; cin>>maxv; for(int i = 1; i < n; i++){ cin>>x; maxv = max(maxv, x); } cout<<maxv<<endl; return 0; }
|
L.看错题
题目描述:
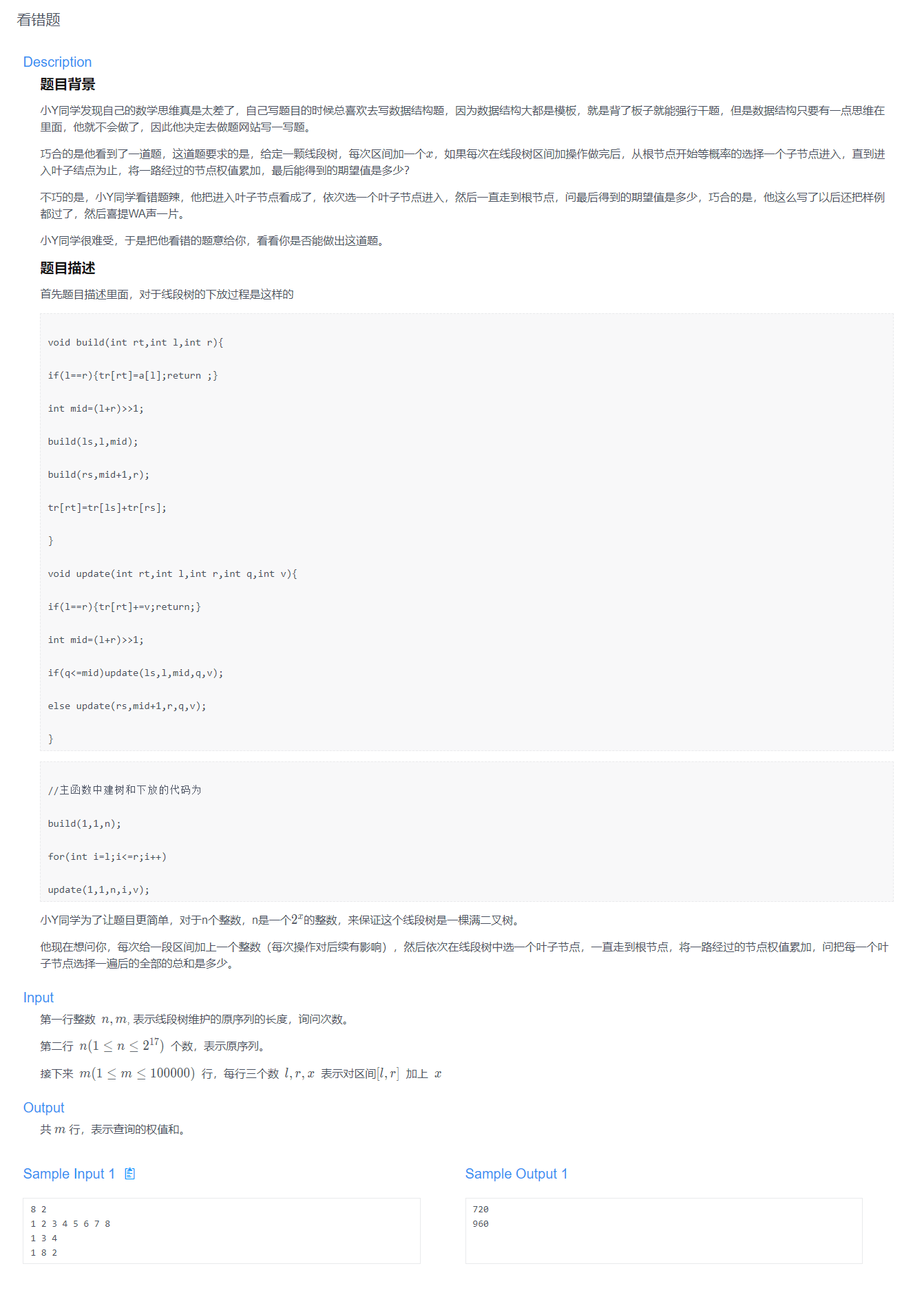
解题思路:
每个原序列的值对整个树权值的贡献度都为$gx = 1 + 2 + 2^2 + 2 ^3 + …+2^x$。故树权值为序列和$(val)$乘上贡献度。再序列更新时只需要令序列和加上区间内元素个数乘增加的权值,然后重新计算树权值。
代码展示:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28
| #include <bits/stdc++.h> using namespace std; typedef long long ll; const int mod=1e9+7; const int inf=0x7f7f7f7f; const int maxn=1e6+50;
ll n, m, val = 0, gx = 0, l, r, x;
int main(){ scanf("%lld%lld", &n, &m); for(ll i = 1; i <= n; i *= 2){ gx += i; } for(int i = 0; i < n; i++){ scanf("%lld", x); val += x; }
for(int i = 0; i < m; i++){ scanf("%lld%lld%lld", &l, &r, &x); val += (r - l + 1) * x; ll res = val * gx; printf("%lld\n",res); } return 0; }
|
做题情况:
AC 六题
排名:662 / 4579
总结反思:
F被卡精度了没写出来。